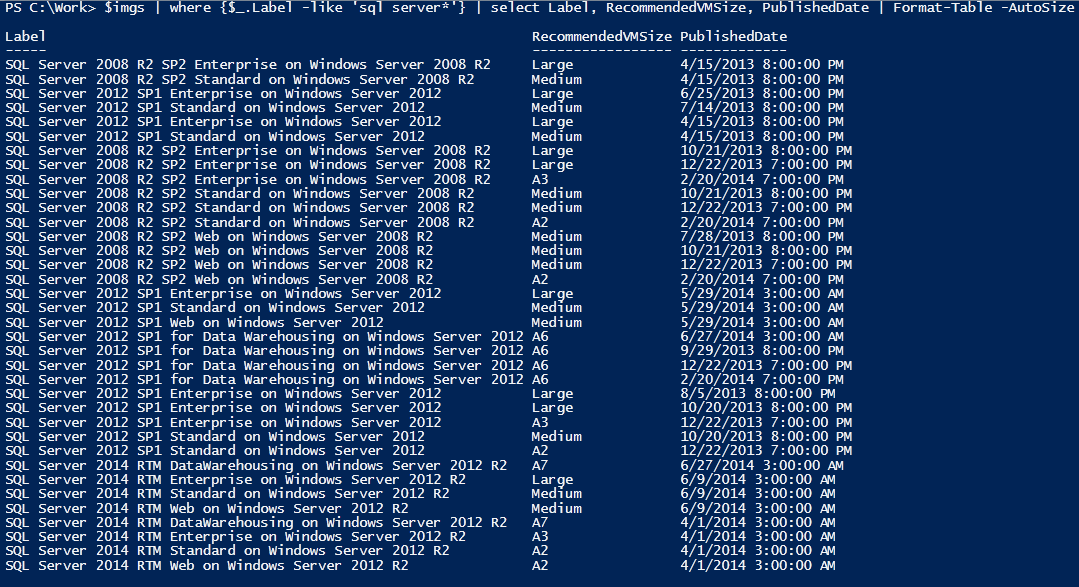
I have revisited this issue here:
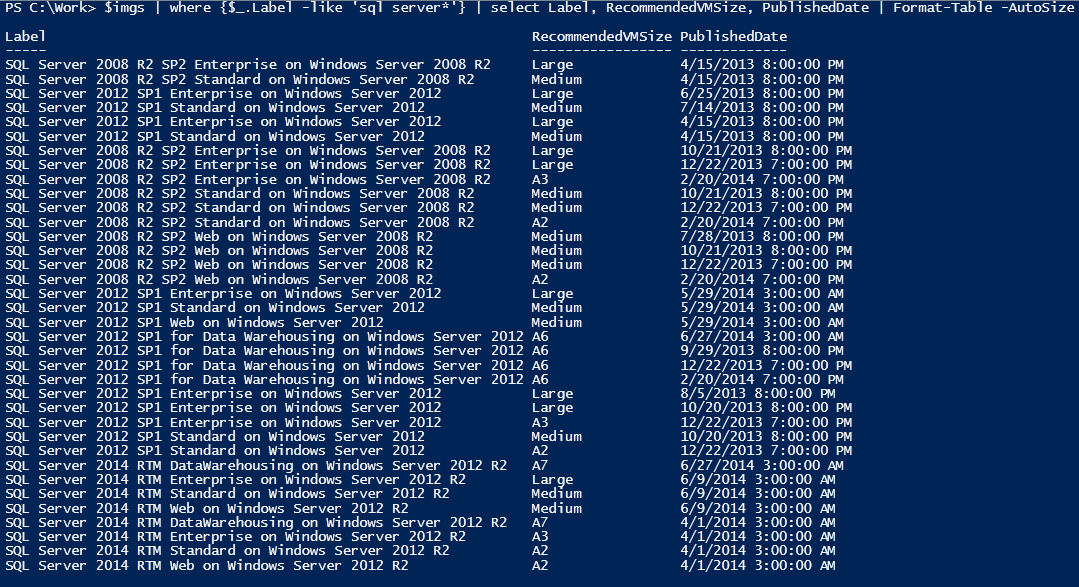
Here is a simple hardware audit using the RedFish API that is part of Dell iDrac. This is a phase 1 of what will be a far more useful estate wide audit function that I am working on.
The code return a small summary of the data it collects to the screen. If you want more then have a look what is stored in this variable:
$serveridracinfoJSON
As usual, please reach out to me if you have questions/edits, or leave something in the comments.
# Name : iDrac hardware Audit V1.0
# Author : Matt Grumpy.tech
# Usage: Add the username/pass and IP of the idrac and run
$Username = "idracusername"
$password = "idracpassword"
$iDrac = "iDrac IP"
# Allow all SSL certificates
add-type @"
using System.Net;
using System.Security.Cryptography.X509Certificates;
public class TrustAllCertsPolicy : ICertificatePolicy {
public bool CheckValidationResult(
ServicePoint srvPoint, X509Certificate certificate,
WebRequest request, int certificateProblem) {
return true;
}
}
"@
[System.Net.ServicePointManager]::CertificatePolicy = New-Object TrustAllCertsPolicy
[System.Net.ServicePointManager]::SecurityProtocol = [System.Net.SecurityProtocolType]::Tls12 -bor [System.Net.SecurityProtocolType]::Tls11
##function to aquire the Auth ID & the session ID
function Get-AuthIDRedfish
{
param ($Username,$password,$iDracBaseUrl)
#iDRAC9 - Login Session
$sessionsUrl = "$iDracBaseUrl/redfish/v1/Sessions"
$credBody = @{'UserName' = $username; 'Password'=$password} | ConvertTo-Json
$headers = @{'Accept' = 'application/json'}
$session = Invoke-WebRequest -Uri $sessionsUrl -Method Post -Body $credBody -ContentType 'application/json' -Headers $headers
$killsessionID = $session.Headers.Location
$authHeaders = @{
'X-Auth-Token' = $session.Headers.'X-Auth-Token'
accept = 'application/json'
}
return $authHeaders, $killsessionID
}
#Get the system inventory from the API and close the serssion
function get-idracInfo
{
param ($Username,$password,$iDracBaseUrl)
$AuthHeaders = Get-AuthIDRedfish -Username $Username -password $password -iDracBaseUrl $iDrac
$SysinfoFullPath = "$iDrac/redfish/v1/Systems/System.Embedded.1"
$SysinfoRAW = Invoke-WebRequest -Uri $SysinfoFullPath -Method Get -Headers $authHeaders[0] -ContentType 'application/json'
$SysinfoJSON = $SysinfoRAW | ConvertFrom-Json
$killsessionpath = $iDrac + $AuthHeaders[1]
Invoke-WebRequest -Uri $killsessionpath -Method Delete -Headers $authHeaders[0] -ContentType 'application/json'
return $SysinfoJSON
}
$serveridracinfoJSON = get-idracInfo -Username $Username -password $password -iDracBaseUrl $iDrac
#Code to translate some values from JSON and display to screen
$SHName = $serveridracinfoJSON.Hostname
$BVersion = $serveridracinfoJSON.BiosVersion
$SVersion = $serveridracinfoJSON.Model
$TotMem = $serveridracinfoJSON.memorysummary.TotalSystemMemoryGiB
$ServiceTag = $serveridracinfoJSON.sku
$ProcCount = $serveridracinfoJSON.ProcessorSummary.Count
$ProcModel = $serveridracinfoJSON.processorsummary.model
$LogicalCoreCount = $serveridracinfoJSON.ProcessorSummary.LogicalProcessorCount
Clear
Write-Output "Idrac IP : $idrac "
Write-Output "Server hostname : $SHName "
Write-Output "CPU Count : $ProcCount "
Write-Output "CPU Model : $ProcModel "
Write-Output "Thread count : $LogicalCoreCount "
Write-Output "Total Memory : $TotMem "
Write-Output "Bios Version : $BVersion "
Write-Output "Server Model : $SVersion "
Write-Output "Service Tag : $ServiceTag "