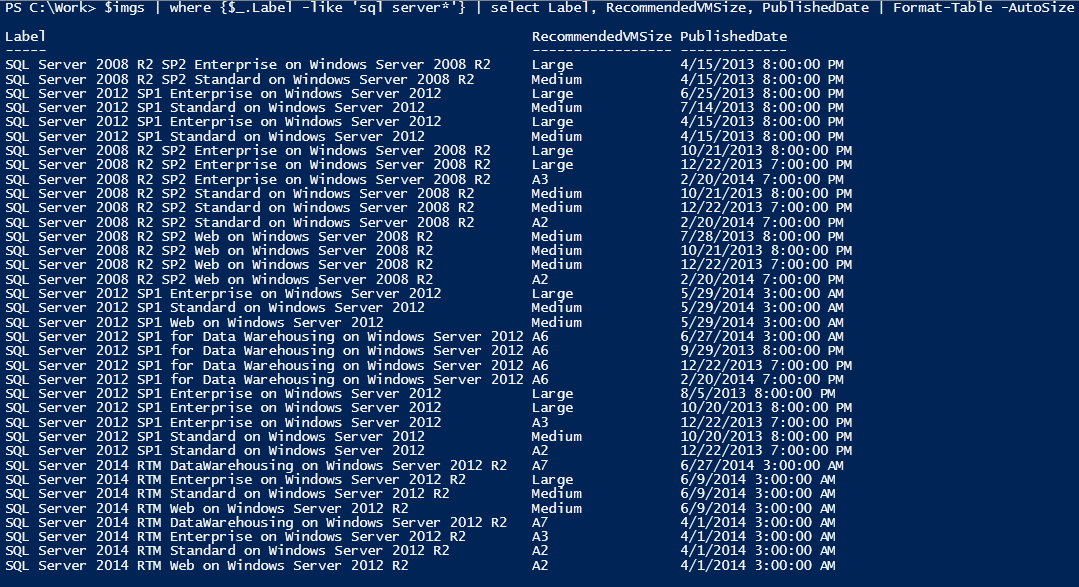
I am currently working on what should be a fun little script that will eventually make its way on to here. The parts of this script are quite useful on there own so I will upload a page on each as I write them.
We are working with the API for the domain provider www.openprovider.eu the guide for this can be found here.
This API uses something called Bearer authentication. You authenticate once with your username and password and are given a bearer code. You then use that for the rest of the API actions.
This is what it looks like in PowerShell:
function Get-BearereCreds
{
$EndPoint = "https://api.openprovider.eu/v1beta/auth/login"
##Configure Payload
$Body = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$Body.Add("ip","0.0.0.0")
$Body.Add("username","UsernameHere")
$Body.Add("password","PasswordPlease")
$Body = $Body | ConvertTo-Json
$Bearer = (Invoke-RestMethod -Method Post -Uri $EndPoint -body $Body -ContentType 'application/json' ).data
return $Bearer
}
$Bearer = Get-BearereCreds
It's important to put these requests into functions as the bearer code expires after a set time, meaning it we want to perform scheduled tasks or a task that takes a long time we need to periodically request a new one.