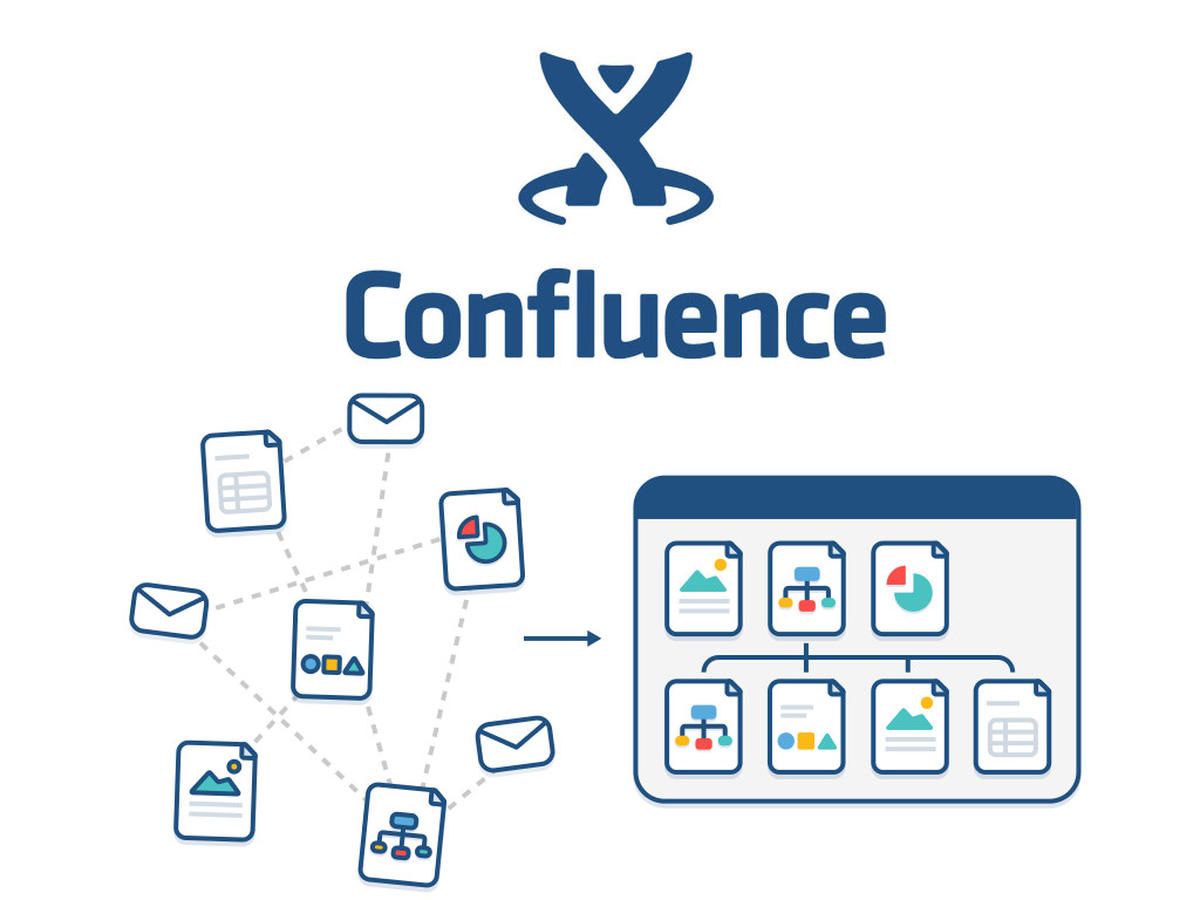
Something a with a little more meat on it for those of you that have used Atlassian Confluence.
I imagine this would usually be done in Python or PHP. If you are a Windows engineer and you understand PowerShell that can be an obsticle.
This basic script will allow you to read an exsisting Confluence page, edit the content and then re-upload the page, obtaining a new version number in the process.
This is very much a first version of this, it will be expanded.
##Configure headers
$Headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$Headers.Add("Authorization","Basic R3J1bXB0ZWNoOkdydW1weXBhc3N3b3Jk") ##This is your username and password encoder in Base64.
$Headers.add("Content-Type","application/json")
$Headers.add("Accept","application/json")
## The API endpoint
$APIEndPoint = "https://Confluence.url.co.uk/confluence/rest/api/content/42598424?expand=body.storage,version" ## You need to have the exapand part of this for it to work.
##Bypass certificate errors
add-type @"
using System.Net;
using System.Security.Cryptography.X509Certificates;
public class TrustAllCertsPolicy : ICertificatePolicy {
public bool CheckValidationResult(
ServicePoint srvPoint, X509Certificate certificate,
WebRequest request, int certificateProblem) {
return true;
}
}
"@
[System.Net.ServicePointManager]::CertificatePolicy = New-Object TrustAllCertsPolicy
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Ssl3, [Net.SecurityProtocolType]::Tls, [Net.SecurityProtocolType]::Tls11, [Net.SecurityProtocolType]::Tls12
#This is the body. This should be HTML.
$newdata = 'This is data'
##This gets the page as it currently is, you need this for things like the version numbers
$CurrentPage = Invoke-RestMethod $APIEndPoint -Method get -Headers $Headers
##increment the version number
$NewVersion = ($CurrentPage.version.number + 1 )
##create the payload containing the new data
$Body = '{"type":"' + $CurrentPage.type + '","title":"' + $CurrentPage.title + '", "version":{"number":'+ $NewVersion + '},"body":{"storage":{"value":"' + $newdata + '","representation":"storage"}}}'
##Create a new version of the page and upload the data
Invoke-RestMethod https://Confluence.url.co.uk/confluence/rest/api/content/42598424?expand=body.storage -Method Put -Headers $Headers -Body $body