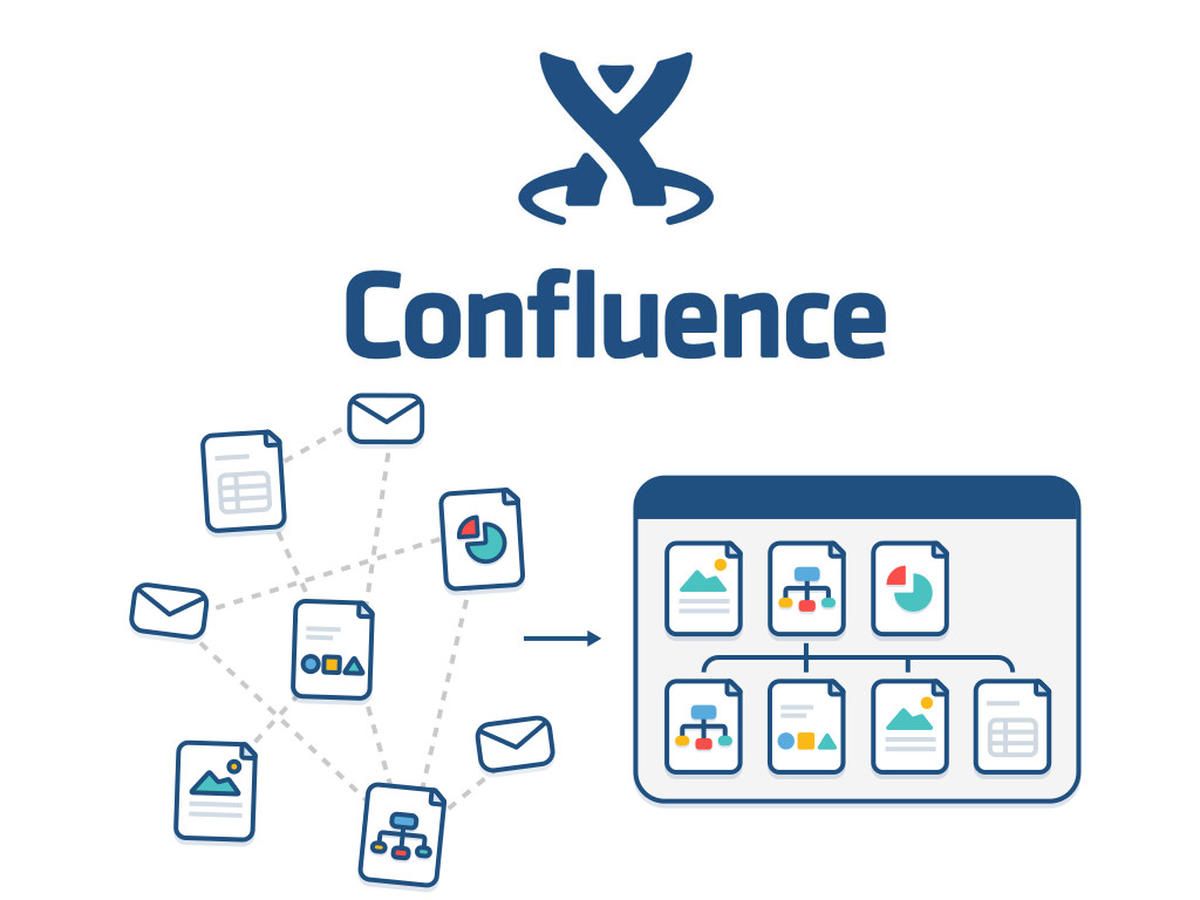
Ok here is a big one for you. Its in functions so you should be able to split it out and use the bits you need pretty easily.
This does a basic machine audit and then uploads the information into a table on a confluence page. It also creates a new page for every server audited.
Im not going to explain it in too much detail. If you need more help please get in touch.
$ServerNames = 'Server1','Server2' #You could (should) get this from AD
$SpaceID = 'ID' #The ID of confluence space (mine just happens to be called ID
function Bypass-CertErrors # you may have gone to the trouble to do this properly. I haven't
{
##Bypass certificate errors
add-type @"
using System.Net;
using System.Security.Cryptography.X509Certificates;
public class TrustAllCertsPolicy : ICertificatePolicy {
public bool CheckValidationResult(
ServicePoint srvPoint, X509Certificate certificate,
WebRequest request, int certificateProblem) {
return true;
}
}
"@
[System.Net.ServicePointManager]::CertificatePolicy = New-Object TrustAllCertsPolicy
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Ssl3, [Net.SecurityProtocolType]::Tls, [Net.SecurityProtocolType]::Tls11, [Net.SecurityProtocolType]::Tls12
}
function Get-ConfHeaders #A function to get a confluence page header information
{
##Configure headers
$Headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$Headers.Add("Authorization","Basic U2NyaXB0bzpAOTprfmsicycmS2dkyyyy==") ##This is your username and password encoded in Base64.
$Headers.add("Content-Type","application/json")
$Headers.add("Accept","application/json")
return $Headers
}
function Check-PageExsists #Check if a page already exsists before creating it
{
param( $ServerName, $SpaceID )
Bypass-CertErrors
$headers = Get-ConfHeaders
## The API endpoint
$APIEndPoint = "https://Confluence.domain.co.uk/confluence/rest/api/"
$ServerPage = "content?title=" + $serverName + "&spaceKey=" + $SpaceID + "&expand=history"
$FullServerLink = $APIEndPoint + $ServerPage
##check if the page exsists
$CheckPage = (Invoke-RestMethod $FullServerLink -Method get -Headers $Headers).results.title
If ($CheckPage -eq $ServerName)
{$PageStatus = $true}
else {$PageStatus = $false}
return $PageStatus
}
function Create-NewConfPage # Create a new page
{
Param ($ServerName,$SpaceID,$Data)
Bypass-CertErrors
$headers = Get-ConfHeaders
## The API endpoint
$APIEndPoint = "https://Confluence.domain.co.uk/confluence/rest/api/"
$ServerPage = "content?title=" + $serverName + "&spaceKey=" + $SpaceID + "&expand=history"
$FullServerLink = $APIEndPoint + $ServerPage
$Body = '{"type":"page","title":"' + $ServerName + '", "ancestors":[{"id":35685070}],"space":{"key":"ID"},"body":{"storage":{"value":"' + $Data + '","representation":"storage"}}}' # the ID part of this is the parent page. If there isnt a parent just leave it blank
Invoke-RestMethod $FullServerLink -Method Post -Headers $Headers -Body $Body
}
function Get-ServerInfo #Collect some basic server information
{
Param ($ServerName)
$parameters = @{computername=$ServerName}
Invoke-Command -ScriptBlock {
$IPV4Regex = '(?:(?:1\d\d|2[0-5][0-5]|2[0-4]\d|0?[1-9]\d|0?0?\d)\.){3}(?:1\d\d|2[0-5][0-5]|2[0-4]\d|0?[1-9]\d|0?0?\d)'
$ServerDeets = @{}
$ComputerSystem = Get-WmiObject -Class Win32_ComputerSystem
If ($ComputerSystem.model -eq "Virtual Machine")
{
$IsVM = $true
$CurrentVMHost = (get-item "HKLM:\SOFTWARE\Microsoft\Virtual Machine\Guest\Parameters").GetValue("HostName")
$ServerDeets.Add('System Type',"Virtual Machine")
$serialNumber = "N/A"
$IPMIAddress = "Hosted by " + $CurrentVMHost
}
Else{ $isVM = $false}
if ($IsVM -eq $false)
{
$IPMIfull = racadm getniccfg
$IPMIAddress = ([regex]::Matches($IPMIfull, $IPV4Regex)).value | select -first 1
$serialNumber = Get-CimInstance Win32_Bios | Select-Object SerialNumber -ExpandProperty SerialNumber
}
$hostname = hostname
$FullIPAddress = Resolve-DnsName -Type A $hostname | Where-Object {$_.IPAddress -like "172.*"} | Select-Object IPaddress -ExpandProperty IPaddress
$FullSystemInfo = Get-WmiObject -Class Win32_ComputerSystem
$MoreSystemInfo = Get-WMIObject win32_operatingsystem
$ServerDeets.Add('IP Addresses',$FullIpAddress)
$ServerDeets.Add('IPMI Details',$IPMIAddress)
$ServerDeets.Add('OS',$MoreSystemInfo.Caption)
$ServerDeets.Add('Serial Number',$serialNumber)
$ServerDeets.Add('Manufacturer',$FullSystemInfo.Manufacturer)
$ServerDeets.Add('Model',$FullSystemInfo.Model)
return $ServerDeets
}@parameters
}
Foreach ($ServerName in $ServerNames)
{
$PrePageCheck = Check-PageExsists -ServerName $ServerName -SpaceID $SpaceID
if ($PrePageCheck -ne $true)
{
$serverDeets = Get-ServerInfo -ServerName $ServerName
$serverDeetsHTML = $serverDeets.GetEnumerator()| select Key,Value | ConvertTo-Html -Fragment
Create-NewConfPage -ServerName $ServerName -SpaceID $SpaceID -Data $ServerDeetsHTML
}
}